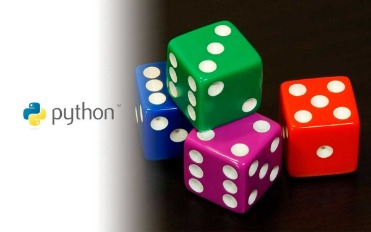
Content
Using Python language and libraries like numpy and scipy, you can simply work wonders in data science, as shown in this task. When you give it a 2d array, the NumPy exponential function simply computes for every input value x in the input array, and returns the result in the form of a NumPy array. The numpy.exp function will take each input value, , and apply it as the exponent to the base .
Before we get into the specifics of the numpy.exp function, let’s quickly review NumPy. Calculating the power of a number is a common mathematical operation. For instance, if you’re creating a program that helps students in a sixth-grade math class revise powers, you would need a power function. Write a Python program to get the square root and exponential of a given decimal number.
The exp() function in Python allows users to calculate the exponential value with the base set to e. We have the function called exp() in the math module which uses the value of e as base. The math.pow() function always returns a float value whereas in the pow() function we are getting int values most of the time.
The math module also has its own implementation of pow() for the same purpose. In the pow() function, we can pass the base value and the exponent value, these values can be of different data types including integers, float, and complex. Here we iterate through the loop many times to calculate the final value. But we have simpler methods for calculating the exponential value in python.
We publish tutorials about NumPy, Pandas, matplotlib, and data science in Python. For more information, read our fantastic tutorial about NumPy exponential. Now, let’s compute for each of these values using numpy.exp. I want to show you this to reinforce the fact that numpy.exp can operate on Python lists, NumPy arrays, and any other array-like structure. As you can see, this NumPy array has the exact same values as the Python list in the previous section. Ok, we’re basically going to use the Python list as the input to the x argument. To be clear, this is essentially identical to using a 1-dimensional NumPy array as an input.
However, I think that it’s easier to understand if we just use a Python list of numbers. Here, I’ll show you a few examples of how to use numpy.exp. For example, there are tools for calculating summary statistics. NumPy has functions for calculating means of a NumPy array, calculating maxima and minima, etcetera. In addition to providing functions to create NumPy arrays, NumPy also provides tools for manipulating and working with NumPy arrays. NumPy is essentially a Python module that deals with arrays of numeric data. You can think of these arrays like row-and-column structures, or like matrices from linear algebra.
Browse other questions tagged python math operators or ask your own question. The most obvious thing to note is math.pow() resulted in an OverflowError. This means that math.pow() can’t support large-valued exponents, most likely because of the way that this module has implemented exponentiation. Both these functions have 2 arguments, the first argument is for the base number, and the second is for the exponent.
Oops, You will need to install Grepper and log-in to perform this action. ‘pip’ is not recognized as an internal or external command, operable program or batch file. Write a Python function to check whether a number is in a given range. Identify the module required to be included for the exp() work in a Python code. Hyperbolic functionsare analogs of trigonometric functions that are based on hyperbolas instead of circles. Improved the algorithm’s accuracy so that the maximum error is under 1 ulp .
Python Power: A Step
As the pow() function first converts its argument into float and then calculates the power, we can see some differences in return type. This is the simplest method for calculating the exponential value in python. Loops will help us execute the block of code, again and again, to take its benefit for calculating the exponential value in python. In this article, we will learn about calculating the exponential value in Python using different ways, but first, let’s understand its mathematical concept. We have declared three variables and assigned values with different numeric data types to them. We have then passed them to the exp() method to calculate their exponents.
The library comes installed in Python, hence you are not required to perform any additional installation in order to be able to use it. For more info you can find the official documentation here. In the above example, the integer 3 has been coerced to 3.0, a float, for addition operation and the result is also a float. In this section, we will explore the Math library functions used to find different types of exponents and logarithms.
The mathematical concept of a function expresses an intuitive idea of how one value completely determines the value of another value. In today’s world, the importance of conducting data science research is gaining momentum every day. This applies to so many aspects of the life of an individual, and of society as a whole. Accurate modeling of social, economic, and natural processes is vital.
If we apply an exponential function and a data set x and y to the input of this method, then we can find the right exponent for approximation. The math.pow() function comes from the math module and it is the fastest way to calculate the exponential value with time complexity O. Apart from the built-in pow() function, we have the math.pow() function which comes from the math module in python which contains some useful mathematical functions. To fit an arbitrary curve we must first define it as a function. We can then call scipy.optimize.curve_fit which will tweak the arguments to best fit the data. In this example we will use a single exponential decay function.
Program To Plotting Exponential Function?
And as you saw earlier in this tutorial, the np.exp function works with both scalars and arrays. Finally, let’s use the numpy.exp function with a 2-dimensional array.
- We have then passed them to the exp() method to calculate their exponents.
- In Math, the exponent is referred to the number of times a number is multiplied by itself.
- Or, in other words, the number 5 is multiplied by itself 3 times.
- Finally, let’s use the numpy.exp function with a 2-dimensional array.
- If we apply an exponential function and a data set x and y to the input of this method, then we can find the right exponent for approximation.
James Gallagher is a self-taught programmer and the technical content manager at Career Karma. He has experience in range of programming languages and extensive expertise in Python, HTML, CSS, and JavaScript.
Character Sets
To do this, we will use the standard set from Python, the numpy library, the mathematical method from the sсipy library, and the matplotlib charting library. For example, take data that describes the exponential increase in the spread of the virus. This data can be approximated fairly accurately by an exponential function, at least in pieces along the X-axis. One of the important processes in data analysis is the approximation process. If you correctly approximate the available data, then it becomes possible to estimate and predict future values. Thus, a weather forecast, a preliminary estimate of oil prices, economic development, social processes in society, and so on can be made.
The function takes only one argument num of which we want to find exponential. The number to be multiplied by itself is called thebase,and the number of times it is to be multiplied is theexponent. There, you can see pow returned 8.0 i.e. it converted both int arguments to float. In Python, you may use different ways for calculating the exponents. If provided, it must have a shape that the inputs broadcast to. If not provided or None, a freshly-allocated array is returned. ¶Calculate the exponential of all elements in the input array.
We’ll create a 2-d array using numpy.arange, which we will reshape into a 2-d form with the NumPy reshape method. I just want to point this out, because in this tutorial I’m referring to NumPy as np. That will only work properly though if you import NumPy with the code import numpy as np. If the first two arguments are specified, the stated base to the power of the exponent is calculated.
It is advisable to use pow instead of pow%2 because the efficiency is more here to calculate the modulo of the exponential value. Although Python doesn’t use the method of squaring but still shows complexity due to exponential increase with big values. There are multiple ways for calculating the exponential value in Python. The Python Math Library provides us with functions and constants that we can use to perform arithmetic and trigonometric operations in Python.
This is a good shorthand that makes your code a little simpler and faster to write. Technically, this input will accept NumPy arrays, but also single numbers or array-like objects. So you can actually use Python lists and other array-like objects as inputs to the x parameter. Essentially, you call the function with the code np.exp() and then inside of the parenthesis is a parameter that enables you to provide the inputs to the function. So you can use NumPy to change the shape of a NumPy array, or to concatenate two NumPy arrays together.
Using Exponents In Python
Note that when you convert a value to an int in this way, it will be truncated rather than being rounded off. We can use the calculated parameters to extend this curve to any position by passing X values of interest into the function we used during the fit. Fitting an exponential curve to data is a common task and in this example we’ll use Python and SciPy to determine parameters for a curve fitted to arbitrary X/Y points. You can follow along using the fit.ipynb Jupyter notebook. The Python language allows users to calculate the exponential value of a number in multiple ways. If we need to find the exponential of a given array or list, the code is mentioned below. Hi, guys today we have got a very easy topic i.e exponential function in Numpy – Python.